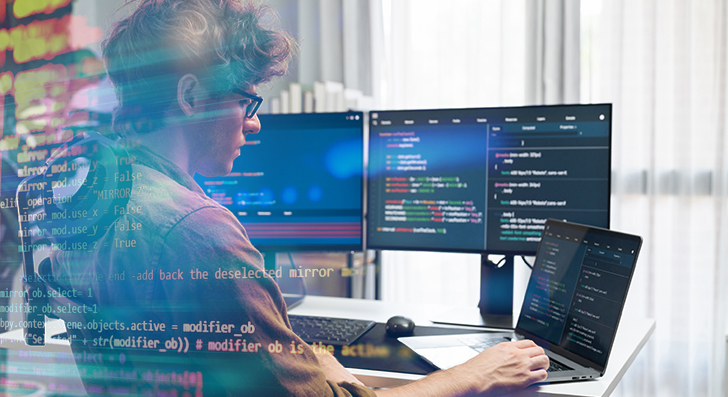
Scalability usually means your application can deal with growth—extra end users, more details, plus more website traffic—with no breaking. As being a developer, building with scalability in your mind saves time and strain afterwards. Listed here’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really anything you bolt on later—it should be part of your respective strategy from the start. Numerous apps fail if they improve quick mainly because the original style and design can’t deal with the additional load. As a developer, you must think early regarding how your method will behave stressed.
Start by developing your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Alternatively, use modular design or microservices. These patterns split your application into smaller, independent areas. Each module or services can scale on its own without impacting The full procedure.
Also, consider your database from day just one. Will it need to handle 1,000,000 buyers or just a hundred? Choose the appropriate form—relational or NoSQL—dependant on how your data will develop. Prepare for sharding, indexing, and backups early, Even when you don’t need them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under present problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that assistance scaling, like concept queues or celebration-pushed programs. These support your app deal with much more requests with out obtaining overloaded.
Whenever you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing foreseeable future head aches. A nicely-prepared method is easier to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Databases
Selecting the right databases is a vital Component of constructing scalable programs. Not all databases are built a similar, and utilizing the Improper one can gradual you down or maybe bring about failures as your app grows.
Begin by understanding your details. Could it be extremely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a good in good shape. These are typically robust with interactions, transactions, and consistency. In addition they assist scaling methods like browse replicas, indexing, and partitioning to deal with more visitors and facts.
When your information is a lot more versatile—like person activity logs, products catalogs, or documents—look at a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your examine and create designs. Have you been executing lots of reads with less writes? Use caching and browse replicas. Are you handling a weighty generate load? Consider databases that could tackle substantial generate throughput, or even occasion-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not need to have Highly developed scaling features now, but choosing a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your info dependant upon your entry designs. And constantly watch databases effectiveness while you increase.
Briefly, the appropriate databases relies on your application’s composition, velocity desires, And just how you assume it to increase. Just take time to choose properly—it’ll conserve a lot of problems later.
Optimize Code and Queries
Speedy code is essential to scalability. As your application grows, every single tiny delay provides up. Improperly published code or unoptimized queries can slow down functionality and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t choose the most advanced Resolution if a simple a person will work. Keep your capabilities limited, focused, and straightforward to test. Use profiling applications to search out bottlenecks—destinations in which your code takes far too lengthy to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual items down greater than the code alone. Make certain Each individual query only asks for the info you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
If you recognize the exact same data currently being asked for again and again, use caching. Retailer the results briefly working with tools like Redis or Memcached which means you don’t should repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which more info load balancing and caching are available in. These two tools help keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to 1 server performing all the do the job, the load balancer routes buyers to unique servers determined by availability. This implies no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused quickly. When people request the identical info all over again—like a product page or maybe a profile—you don’t ought to fetch it in the databases each and every time. You can provide it from the cache.
There's two typical different types of caching:
1. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces databases load, increases pace, and will make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does alter.
Briefly, load balancing and caching are easy but strong tools. Collectively, they assist your application cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application develop very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to invest in hardware or guess foreseeable future ability. When website traffic improves, you could add more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are A further vital Resource. A container deals your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app among environments, from your notebook on the cloud, with no surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If 1 part of your respective app crashes, it restarts it quickly.
Containers also help it become very easy to separate aspects of your app into services. You may update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy simply, and recover speedily when problems come about. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and assist you to keep centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when factors go Incorrect. Checking can help the thing is how your app is executing, place challenges early, and make much better choices as your application grows. It’s a critical part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and response time. These inform you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for significant challenges. One example is, if your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems quick, often right before people even detect.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems till it’s much too late. But with the best tools in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring that it works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Commence compact, Believe massive, and Establish clever.